2월 19일 자 학습 내용 정리입니다.
✅ Mongoose CRUD
Mongoose ODM의 CRUD 관련 함수는 다음과 같다.
CRUD | 함수명 |
CREATE | create |
READ | find findById findOne |
UPDATE | updateOne updateMany findByIdAndUpdate findOneAndUpdate |
DELETE | deleteOne deleteMany findByIdAndDelete findOneAndDelete |
✔️ create
.create() 를 사용하여 Document를 생성한다.
Document Object 혹은 Document Object의 Array를 전달하여 단일, 복수의 Document를 생성할 수 있다.
create 함수의 반환값은 Promise 값으로 생성된 Document이다.
const { Post } = require('./models');
async function Func() {
const created = await Post.create({
title: 'first title',
content: 'first content'
});
const multipleCreated = await Post.create([
{
title: 'first title',
content: 'first content'
},
{
title: 'second title',
content: 'second content'
}
]);
}
✔️ find
.find()로 Document를 검색한다.
query를 사용하여 검색하거나 ObjectID를 사용해 검색할 수 있다.
반환값은 Document Array 혹은 하나의 Document이다.
Model.find({})로 해당 모델의 모든 Document를 가져올 수 있다.
const { Post } = require('./models');
async function Func() {
const listPost = await Post.find(query);
const onePost = await Post.findOne(query);
const postById = await Post.findById(id);
const example = await Post.find({ title: 'first title' });
const allPost = await Post.find({});
}
✔️ update
.update()로 Document를 수정한다.
findByIdAndUpdate와 findOneAndUpdate는 Document를 검색하고 업데이트를 반영하여 수정된 Document를 반환한다.
.update()는 기본적으로 $set operator를 사용하여 Document를 통째로 변경하지 않고 이미 있는 부분에 대한 수정이라면 그 부분만, 없는 부분에 대한 수정이라면 새로운 내용을 추가한다.
const { Post } = require('./models');
async function Func() {
const updateResult = await Post.updateOne(query, {
// 수정 사항
...
});
const postById = await Post.findByIdAndUpdate(id, {
...
});
}
✔️ delete
.delete()로 Document를 삭제한다.
deleteOne, deleteMany는 삭제된 결과를 반환하는 반면 findByIdAndDelete, findOneAndDelete는 검색된 Document를 반환한다.
const { Post } = require('./models');
async function Func() {
const deleteResult = await Post.deleteOne(query);
const deleteResults = await Post.deleteMany(query);
const onePost = await Post.findOneAndDelete(query);
const postById = await Post.findByIdAndDelete(query);
}
✅ query
query란 BSON 형식으로 기본 문법 그대로 mongoose에서 사용이 가능하다.
SQL의 where와 유사한 조건절이다.
const { Person } = require('./models');
Person.find({
name: 'kiki',
age: {
$lt: 20,
$gte: 10,
},
languages: {
$in: ['ko','en'],
},
$or: [
{ status: 'ACTIVE' },
{ isFresh: true },
]
})
자주 사용하는 쿼리들은 다음과 같다.
- { key, value } : exact match
- $lt, $lte, $gt, $gte : range query
- lt : little(<)
- lte : little or equal(<=)
- gt : greater(>)
- gte : greater or equal(>=)
- $in : 다중 값 검색
- $or : 다중 조건 검색
참고로 Mongoose는 query로 배열이 주어지면 자동으로 $in query를 생성한다.
따라서 아래 두 find()의 결과는 동일하다.
const { Person } = require('./models');
Person.find({
name: ['kiki', 'didi']
});
Person.find({
name: {
$in: ['kiki', 'didi']
}
})
query operator에 대한 자세한 내용은 다음 링크에서 확인할 수 있다.
https://docs.mongodb.com/manual/reference/operator/query/
Query and Projection Operators — MongoDB Manual
Docs Home → MongoDB ManualFor details on specific operator, including syntax and examples, click on the specific operator to go to its reference page.For comparison of different BSON type values, see the specified BSON comparison order.NameDescriptionMat
docs.mongodb.com
✅ populate
.populate()는 어떠한 document 안에 다른 document를 담지 않고 ObjectID를 사용해 가져오고자 하는 document를 참조하여 사용할 수 있는 방법을 제공한다.
document에는 참조되는 document의 ObjectID를 담고 사용할 때 populate하여 하위 document처럼 사용할 수 있게 해준다.
const { Schema } = require('mongoose');
const Post = new Schema({
user: {
type: Schema.Types.ObjectId,
ref: 'User'
},
comments: [{
type: Schema.Types.ObjectId,
ref: 'Comment'
}]
});
const post = await Post.find().populate(['user', 'comments']);
✅ Express.js + Mongoose ODM
Express.js는 프로젝트 구조를 자유롭게 구성할 수 있기 때문에 어느 부분에 Mongoose ODM을 위치시키면 좋을지 적절한 위치를 결정하는 것이 중요하다.
일반적으로 models 디렉토리에 Schema와 Model을 같이 위치시킨다.
app 객체는 어플리케이션 시작을 의미하는 부분이므로 해당 부분에 데이터베이스 연결을 명시하는 mongoose.connect를 작성한다.
또한 Express.js 어플리케이션은 종료되지 않고 동작하는 웹 서비스이기 때문에 계속해서 데이터베이스가 정상적으로 동작하는지를 파악하기 위해 동작 중 발생하는 데이터베이스 연결 관련 이벤트에 대한 처리를 하는 것이 좋다.
Mongoose ODM connection event는 다음과 같다.
mongoose.connect('----');
mongoose.connection.on('connected', () => {
});
mongoose.connection.on('disconnected', () => {
});
mongoose.connection.on('reconnected', () => {
});
mongoose.connection.on('reconnectFailed', () => {
});
- connected : 연결 완료
- disconnected : 연결 끊김
- reconnected : 재연결 완료
- reconnectedFailed : 재연결 시도 횟수 초과
🔍 참조
create https://mongoosejs.com/docs/api.html#model_Model.create
find https://mongoosejs.com/docs/api.html#model_Model.find
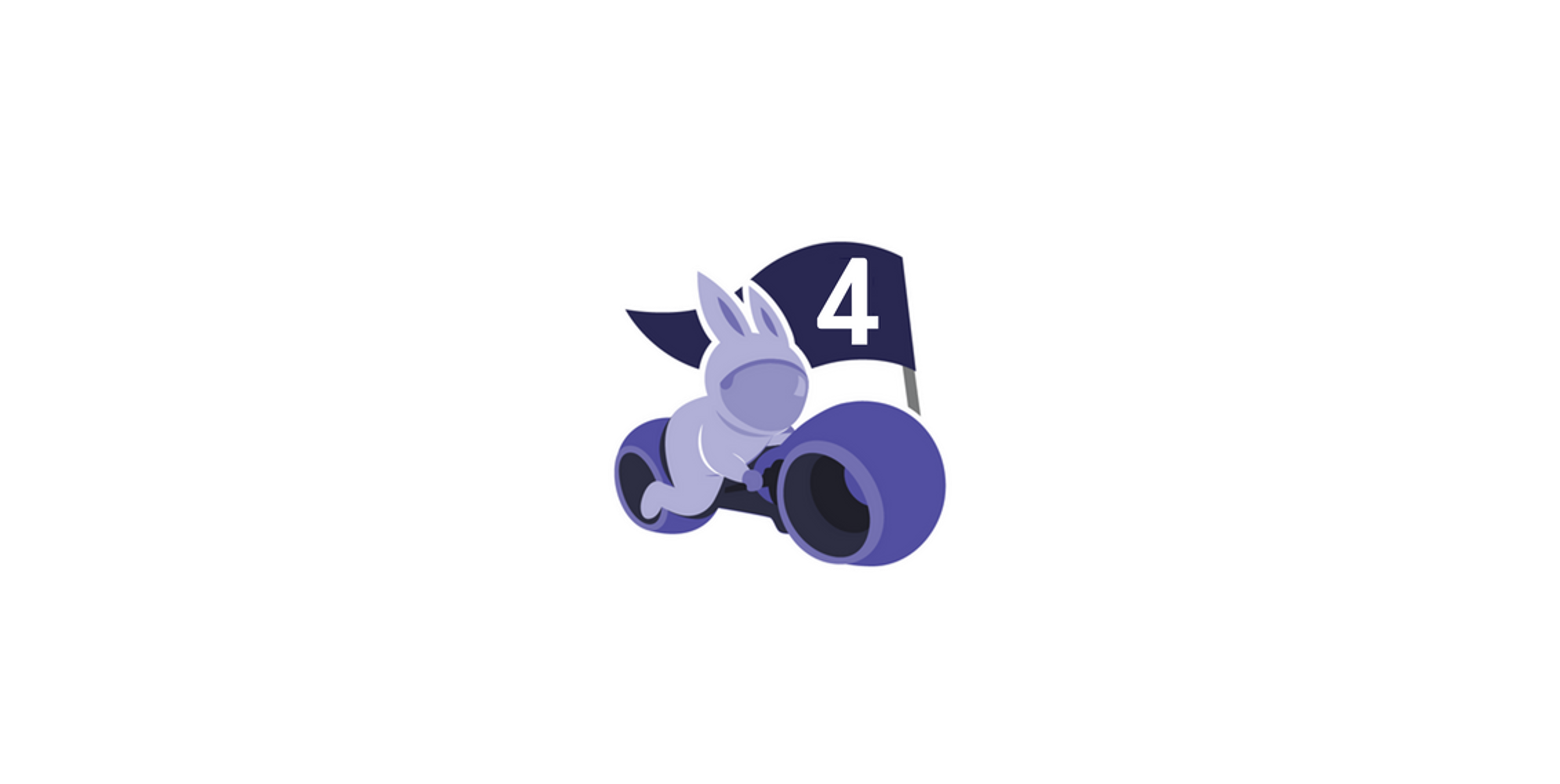
'엘리스 AI 트랙 4기 > elice AI track' 카테고리의 다른 글
[5주차] Express.js + Mongoose로 Pagination 구현하기 (0) | 2022.02.19 |
---|---|
[5주차] Pug와 Async Request Handler, PM2 (0) | 2022.02.19 |
[5주차] Mongoose ODM - (1) (0) | 2022.02.19 |
[5주차] NoSQL과 MongoDB (0) | 2022.02.19 |
[5주차] Express.js의 Middleware와 JSON (0) | 2022.02.18 |
댓글