이수안컴퓨터연구소의 NumPy 한번에 끝내기 영상을 보고 정리한 내용입니다.
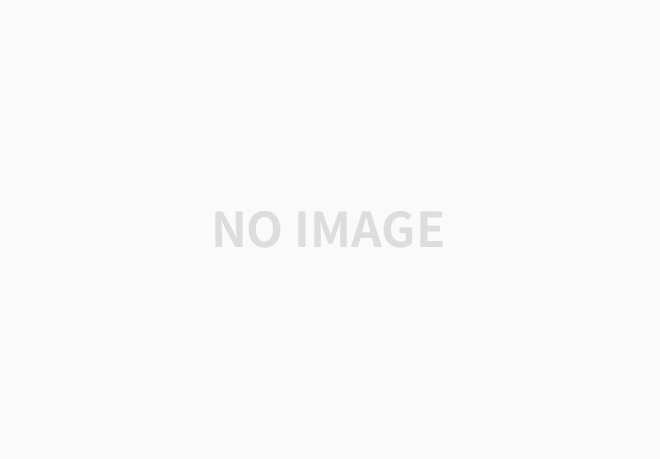
✅ 배열 추가
✔️ numpy.append()
배열의 끝에 값을 추가한다.
numpy.append(arr, values, axis=None)
- arr: 값을 붙일 배열. values는 복사된 arr에 append된다.
- values : 이어붙일 값들. arr과 같은 shape을 가지고 있어야 한다.
- axis : 이어붙일 대상이 될 축.
a2 = np.arange(1, 10).reshape(3, 3)
b2 = np.arange(10,19).reshape(3, 3)
print(a2)
print(b2)
# output
# [[1 2 3]
# [4 5 6]
# [7 8 9]]
# [[10 11 12]
# [13 14 15]
# [16 17 18]]
3x3의 shape을 가진 배열 a2와 b2를 만들었다.
a2에 b2를 이어붙인 결과를 c2라고 할 때 axis의 값에 따라 c2는 다음의 값을 가진다.
# axis 지정을 하지 않았을 때
c2 = np.append(a2, b2)
print(c2)
# output
# [ 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18]
먼저 axis를 지정하지 않았을 때는 1차원 배열 형태로 변형되어 결합된다.
a2를 기준으로 b2를 이어붙였기 때문에 a2의 값들 뒤로 b2의 값들이 결합된 것을 확인할 수 있다.
# axis를 0으로 지정했을 때
c2 = np.append(a2, b2, axis=0)
print(c2)
# output
# [[ 1 2 3]
# [ 4 5 6]
# [ 7 8 9]
# [10 11 12]
# [13 14 15]
# [16 17 18]]
axis를 0으로 지정하면 a2의 아래에 b2가 결합된다.
이때 axis를 0으로 지정하기 위해서는 shape[0]을 제외한 나머지 shape이 같아야 이어붙일 수 있다.
# axis를 1로 지정했을 때
c2 = np.append(a2, b2, axis=1)
print(c2)
# output
# [[ 1 2 3 10 11 12]
# [ 4 5 6 13 14 15]
# [ 7 8 9 16 17 18]]
axis를 1로 지정하면 a2의 횡으로 b2가 결합된다.
axis를 0으로 지정했을 때와 마찬가지로 이 경우에 대해서는 shape[1]을 제외한 나머지 shape이 동일해야 이어붙일 수 있다.
✅ 배열 분할
✔️ numpy.concatenate()
튜플이나 배열의 리스트를 인수로 사용해 배열을 연결한다.
numpy.concatenate((a1, a2, ...), axis=0, out=None, dtype=None, casting="same_kind")
- a1, a2, ... : 이어붙일 일련의 배열
- axis : 이어붙일 축. 기본값은 0
a1 = np.array([1, 3, 5])
b1 = np.array([2, 4, 6])
print(np.concatenate([a1, b1]))
# output
# [1 3 5 2 4 6]
a2 = np.array([[1, 2, 3], [4, 5, 6]])
print(np.concatenate([a2, a2]))
# output
# [[1 2 3]
# [4 5 6]
# [1 2 3]
# [4 5 6]]
axis의 기본값이 0으로 되어있기 때문에 2차원 배열을 이어붙일 경우 axis를 지정하지 않으면 수직으로 배열이 연결된다.
같은 배열에 대해 axis를 1으로 지정한 결과는 다음과 같다.
a2 = np.array([[1, 2, 3], [4, 5, 6]])
print(np.concatenate([a2, a2], axis=1))
# output
# [[1 2 3 1 2 3]
# [4 5 6 4 5 6]]
✔️ numpy.vstack()
수직 스택(vertical stack)으로 1차원적으로 연결한다.
concatenate에서 axis를 지정하지 않은 것(혹은 axis를 0으로 지정한 것)과 같다.
numpy.vstack(tup)
- tup : 일련의 ndarray들.
print(np.vstack([a2, a2]))
# output
# [[1 2 3]
# [4 5 6]
# [1 2 3]
# [4 5 6]]
✔️ numpy.hstack()
수평 스택(horizontal stack)으로 2차원적으로 연결한다.
concatenate에서 axis를 1으로 지정한 것과 같다.
numpy.hstack(tup)
- tup : 일련의 ndarray들.
print(np.hstack([a2, a2]))
# output
# [[1 2 3 1 2 3]
# [4 5 6 4 5 6]]
✔️ numpy.dstack()
깊이(depth stack)으로 3차원적으로 연결한다.
즉, 차원을 하나 더 생성한다.
numpy.dstack(tup)
- tup : 일련의 ndarray들.
print(np.dstack([a2, a2]))
# output
# [[[1 1]
# [2 2]
# [3 3]]
#
# [[4 4]
# [5 5]
# [6 6]]]
✔️ numpy.stack()
주어진 배열을 새로운 차원으로 연결한다.
numpy.stack(arrays, axis=0, out=None)
- arrays : 연결할 일련의 배열들.
- axis: array들이 연결될(쌓일) 축. 기본값은 0.
print(np.stack([a2, a2]))
# output
# [[[1 2 3]
# [4 5 6]]
#
# [[1 2 3]
# [4 5 6]]]
✅ 배열 분할
✔️ numpy.split()
주어진 인덱스 혹은 구역 별로 배열을 분할한다.
numpy.split(ary, indices_or_sections, axis=0)
- ary : 분할할 배열.
- indices_or_sections : 분할할 기준점. 정수 혹은 1차원 배열의 값을 가진다.
- 정수의 경우 : 해당 인덱스를 기준으로 삼는다. 나눌 수 없으면 에러를 발생시킨다.
- 1차원 배열의 경우 : 1차원 배열을 값으로 가질 때 해당 배열은 정렬된 상태여야 한다. dimension을 초과하면 그에 따라 sub-array로 빈 배열을 반환한다.
- axis : 분할할 축. 기본값은 0.
a1 = np.arange(0, 10)
print(a1)
b1, c1 = np.split(a1, [5])
print(b1, c1)
b1, c1, d1, e1, f1 = np.split(a1, [2, 4, 6, 8])
print(b1, c1, d1, e1, f1)
# output
# [0 1 2 3 4 5 6 7 8 9]
# [0 1 2 3 4] [5 6 7 8 9]
# [0 1] [2 3] [4 5] [6 7] [8 9]
1차원 배열인 a1을 분할한 결과이다.
인자로 정수값을 주었을 때는 해당 정수값의 인덱스를 기준으로 삼는다.
따라서 5를 주었으니 5번 인덱스인 5를 기준으로 분할되어 b2 = [0 1 2 3 4], c2 = [5 6 7 8 9]의 결과를 얻는다.
[2, 4, 6, 8]처럼 1차원 배열을 인자로 준 경우 각각의 인덱스를 기준으로 자른다.
따라서 b1, c1, d1, e1, f1은 각각 [0 1] [2 3] [4 5] [6 7] [8 9]의 값을 가진다.
✔️ numpy.vsplit()
수직 분할(vertical split)로 1차원으로 분할한다.
numpy.vsplit(ary, indices_or_sections)
a2 = np.arange(1, 10).reshape(3, 3)
print(a2)
b2, c2 = np.vsplit(a2, [2])
print(b2)
print(c2)
# output
# [[1 2 3]
# [4 5 6]
# [7 8 9]]
# [[1 2 3]
# [4 5 6]]
# [[7 8 9]]
✔️ numpy.hsplit()
수평 분할(horizontal split)로 2차원으로 분할한다.
numpy.hsplit(ary, indices_or_sections)
a2 = np.arange(1, 10).reshape(3, 3)
print(a2)
b2, c2 = np.hsplit(a2, [2])
print(b2)
print(c2)
# output
# [[1 2 3]
# [4 5 6]
# [7 8 9]]
# [[1 2]
# [4 5]
# [7 8]]
# [[3]
# [6]
# [9]]
✔️ numpy.dsplit()
깊이 분할(depth split)로 3차원으로 분할한다.
numpy.dsplit(ary, indices_or_sections)
a3 = np.arange(1, 28).reshape(3, 3, 3)
print(a3)
b3, c3 = np.dsplit(a3, [2])
print(b3)
print(c3)
# output
# [[[ 1 2 3]
# [ 4 5 6]
# [ 7 8 9]]
# [[10 11 12]
# [13 14 15]
# [16 17 18]]
# [[19 20 21]
# [22 23 24]
# [25 26 27]]]
# [[[ 1 2]
# [ 4 5]
# [ 7 8]]
# [[10 11]
# [13 14]
# [16 17]]
# [[19 20]
# [22 23]
# [25 26]]]
# [[[ 3]
# [ 6]
# [ 9]]
# [[12]
# [15]
# [18]]
# [[21]
# [24]
# [27]]]
🔍 참조
append https://numpy.org/doc/stable/reference/generated/numpy.append.html
concatenate https://numpy.org/doc/stable/reference/generated/numpy.concatenate.html
vstack https://numpy.org/doc/stable/reference/generated/numpy.vstack.html
hstack https://numpy.org/doc/stable/reference/generated/numpy.hstack.html
dstack https://numpy.org/doc/stable/reference/generated/numpy.dstack.html
stack https://numpy.org/doc/stable/reference/generated/numpy.stack.html
split https://numpy.org/doc/stable/reference/generated/numpy.split.html
vsplit https://numpy.org/doc/stable/reference/generated/numpy.vsplit.html
hsplit https://numpy.org/doc/stable/reference/generated/numpy.hsplit.html
dsplit https://numpy.org/doc/stable/reference/generated/numpy.dsplit.html
'Python > NumPy' 카테고리의 다른 글
[NumPy] 배열 연산 - (1) (0) | 2022.02.05 |
---|---|
[NumPy] 배열 변환 - (1) (0) | 2022.01.24 |
[NumPy] 배열 값 삽입/수정/삭제/복사 (0) | 2022.01.24 |
[NumPy] 인덱싱과 슬라이싱 (0) | 2022.01.23 |
[NumPy] 배열 생성 - (2) (0) | 2022.01.23 |
댓글